JavaScript: The Unavoidable Language
Jul 27, 2020 • posted by Michael Hartl
Learning to code? Learn Enough is based on the idea that you don’t have to learn everything about tech to get started—you just have to learn enough to be dangerous. This is the sixth in a series of posts introducing the main Learn Enough tutorials. You can also read the previous one or the next one. You might also enjoy the Learn Enough JavaScript free sample chapters and free sample videos.
Michael Hartl here from the Rails Tutorial and Learn Enough.
The title of this post is a bit of a dig at JavaScript, a programming language that (perhaps) hundreds love and millions love to hate. This is a bit unfair, though, as JavaScript has grown and matured immeasurably since its development in the mid-1990s. And love it or hate it, everyone agrees that JavaScript is ubiquitous—it’s everywhere because web browsers are everywhere, and JavaScript is the only language that can run inside web browsers.
If you want to learn technical sophistication (Box 1), chances are you’re at least going to have to master the basics of JavaScript. In other words, you’ll have to learn enough JavaScript to be dangerous.
An essential aspect of using computers is the ability to figure things out and troubleshoot on your own, a skill we at Learn Enough call technical sophistication.
Developing technical sophistication means not only following systematic tutorials like Learn Enough JavaScript to Be Dangerous, but also knowing when it’s time to break free of a structured presentation and just start googling around for a solution.
Learning JavaScript can be a rich source of opportunities to practice this important skill. For example, there is a wealth of JavaScript reference material on the Web, but it can be hard to use unless you already know basically what you’re doing. One goal of Learn Enough JavaScript to Be Dangerous is to be the key that unlocks this documentation. This will include lots of pointers to my favorite JavaScript source, the Mozilla Developer Network.
Especially as the exposition gets more advanced, Learn Enough JavaScript to Be Dangerous also frequently includes the exact web searches I used to figure out how to accomplish the particular task at hand. For example, how do you use JavaScript to return all elements on a page that match, say, a particular CSS class? Like this: javascript css class return all elements.
Learn Enough JavaScript
Learn Enough JavaScript to Be Dangerous, which is available as an online course, book, and videos, is designed to provide this essential foundation. It covers the latest JavaScript technologies (including Node.js and ES6) en route to deploying a real interactive website to the live Web. No prior experience with programming is required; the only prerequisites are a familiarity with the command line, text editors, Git, and HTML, such as provided by the corresponding Learn Enough tutorials.
Unlike most JavaScript tutorials, Learn Enough JavaScript treats JavaScript as a general-purpose programming language right from the start, so the examples aren’t confined to the browser. The result is a practical narrative introduction to JavaScript—a perfect complement to both in-browser coding tutorials and the voluminous but hard-to-navigate JavaScript reference material on the Web.
You won’t learn everything there is to know about JavaScript—that would take thousands of pages and centuries of effort—but you will learn enough JavaScript to be dangerous (Figure 1).1
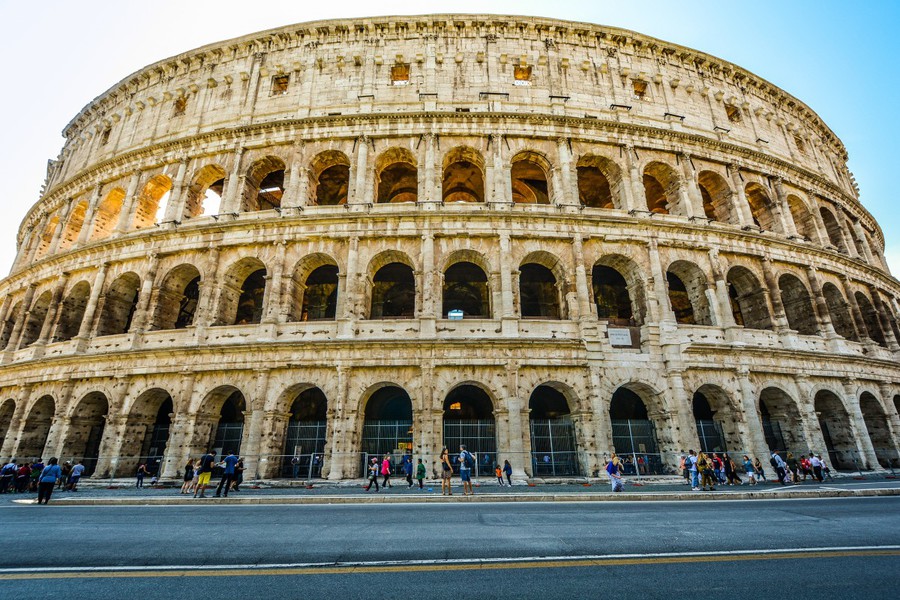
There are no programming prerequisites for Learn Enough JavaScript to Be Dangerous, although it certainly won’t hurt if you’ve programmed before. What is important is that you’ve started developing your technical sophistication (Box 1), either on your own or using the preceding Learn Enough tutorials. These tutorials include the following, which together make for a good list of prerequisites for Learn Enough JavaScript to Be Dangerous:
If you’re up for a challenge…
If you’re up for a challenge, here’s what you’ll get if you join me in following Learn Enough JavaScript to Be Dangerous.
We’ll begin at the beginning with a series of simple “hello, world” programs using several different techniques (Chapter 1), including an introduction to Node.js, a fast and widely used execution environment for JavaScript programs. In line with the Learn Enough philosophy of always doing things “for real”, even as early as Chapter 1 we’ll deploy a (very simple) dynamic JavaScript application to the live Web.
<!DOCTYPE html>
<html>
<head>
<title>Learn Enough JavaScript</title>
<meta charset="utf-8">
<script>
alert("hello, world!");
</script>
</head>
<body>
<h1>Hello, world!</h1>
<p>This page includes an alert written in JavaScript.</p>
</body>
</html>
After mastering “hello, world”, we’ll take a tour of some JavaScript objects, including strings (Chapter 2), arrays (Chapter 3), and other native objects (Chapter 4). Taken together, these chapters constitute a gentle introduction to object-oriented programming with JavaScript.
In Chapter 4, we’ll learn the basics of functions, an essential subject for virtually every programming language. We’ll then apply this knowledge to an elegant and powerful style of coding called functional programming (Chapter 6).
let states = ["Kansas", "Nebraska", "North Dakota", "South Dakota"];
// urls: Imperative version
function imperativeUrls(elements) {
let urls = [];
elements.forEach(function(element) {
urls.push(element.toLowerCase().split(/\s+/).join("-"));
});
return urls;
}
console.log(imperativeUrls(states));
// urls: Functional version
function functionalUrls(elements) {
return elements.map(element => element.toLowerCase().split(/\s+/)
.join('-'));
}
console.log(functionalUrls(states));
Having covered the basics of built-in JavaScript objects, in Chapter 7 we’ll learn how to make objects of our own. In particular, we’ll define an object for a phrase, and then develop a method for determining whether or not the phrase is a palindrome (the same read forward and backward).
Our initial palindrome implementation will be rather rudimentary, but we’ll extend it in Chapter 8 using a powerful technique called test-driven development (TDD). In the process, we’ll learn more about testing generally, as well as how to create a self-contained software package called an NPM module (and thereby join the large and growing ecosystem of software packages managed by npm, the Node Package Manager).
let assert = require("assert");
let Phrase = require("../index.js");
describe("Phrase", function() {
describe("#palindrome", function() {
it("should return false for a non-palindrome", function() {
let nonPalindrome = new Phrase("apple");
assert(!nonPalindrome.palindrome());
});
it("should return true for a plain palindrome", function() {
let plainPalindrome = new Phrase("racecar");
assert(plainPalindrome.palindrome());
});
it("should return true for a mixed-case palindrome");
it("should return true for a palindrome with punctuation");
});
});
In Chapter 9, we’ll apply our new NPM module to a JavaScript web application: a site for detecting palindromes. This will give us a chance to learn about events and DOM manipulation. We’ll start with the simplest possible implementation, and then add several extensions of steadily increasing sophistication, including alerts, prompts, and an example of an HTML form.
In Chapter 10, we’ll learn how to write nontrivial shell scripts using JavaScript, a much-neglected topic that underscores JavaScript’s growing importance as a general-purpose programming language. Examples include reading from both files and URLs, with a final example showing how to manipulate a downloaded file as if it were an HTML web page.
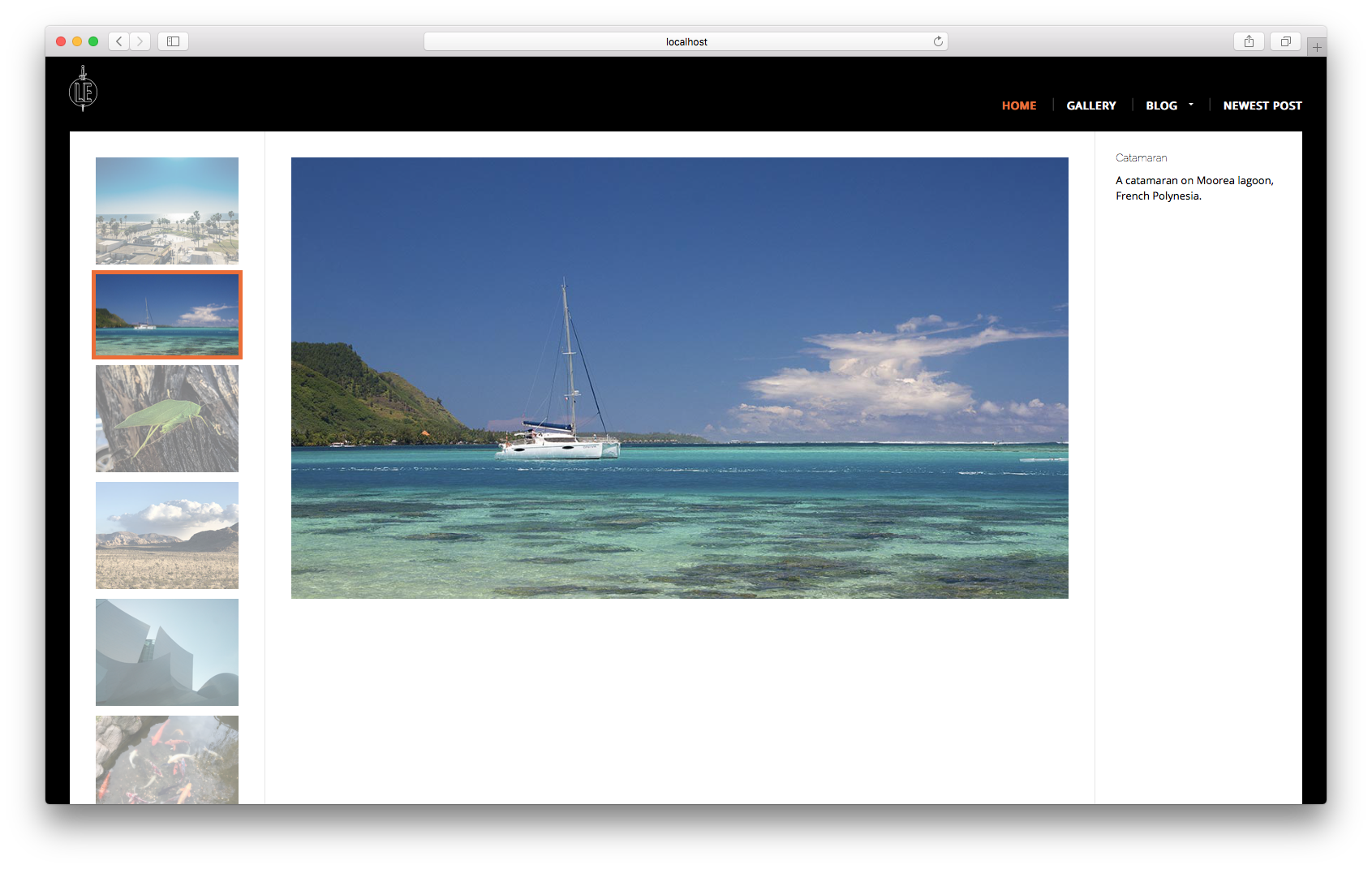
In Chapter 11, we’ll apply the techniques from Chapter 9 and Chapter 10 to a real, industrial-grade website. In particular, we’ll extend the sample application from Learn Enough CSS & Layout to Be Dangerous to add a functional image gallery that dynamically changes images, CSS classes, and page text in response to user clicks. (We’ll be using Git to clone a repository directly, so you’ll be able to build and deploy the image gallery even if you haven’t completed Learn Enough CSS & Layout to Be Dangerous.)
Although full-blown web development with a dynamically rendered front-end and a database back-end is beyond the scope of Learn Enough JavaScript to Be Dangerous, by the end of the book you’ll have a solid foundation on which to build such skills. We’ll end the tutorial with pointers to additional resources for extending your JavaScript knowledge further, as well as to further Learn Enough tutorials for full-stack web development—specifically, using Ruby (via Sinatra) and Ruby on Rails, for which a background in JavaScript is excellent preparation.
How to get it
There are multiple ways to get Learn Enough JavaScript to Be Dangerous. All the different formats cover the same basic material in slightly different ways.
- All Access Subscription: Get full access to the online courses for Learn Enough Command Line, Text Editor, Git, HTML, CSS & Layout, JavaScript, and all the Learn Enough courses for one monthly price. Includes the full 6th edition of the Ruby on Rails Tutorial.
- The Learn Enough JavaScript to Be Dangerous online course subscription or ebook (including three free sample chapters).